Working with ZPL can be cumbersome and frustrating. This is why most ZPL is generated by software applications rather than written by hand. Furthermore, once the ZPL code has been generated, it can be very challenging to make changes to it retroactively.
So, what should you do if you need to make adjustments to a ZPL label? What if you need to rotate it or scale it to make it print properly? What if you need to apply these adjustment(s) to thousands of labels per day?
What NOT to Do
One option would be to convert the ZPL to an image, rotate the image, then convert the image back to ZPL. This solution is far from ideal for the following reasons:
- It typically requires chaining various tools together from multiple vendors.
- It requires more processing power, memory, and network bandwidth.
- It can potentially lead to a loss of print quality, depending on which image formats are used.
- It can cause a reduction in print speed.
A Better Solution
LabelZoom’s web service makes the process of rotating ZPL much simpler. Rather than converting between multiple file formats using multiple tools, you can use a single tool to perform the rotation. Additionally, all the optimizations of native ZPL will be preserved because the label will never be converted to a raster image.
To rotate a label using the LabelZoom API, simply HTTP POST
to the conversion controller using zpl
as both the source and target of the conversion:
POST /api/v2/convert/zpl/to/zpl
Then, you can specify the degree of rotation in a JSON object placed in the query parameter named params
.
Defining the Parameters
First, construct the JSON object for the parameters (rotation must be a multiple of 90°):
{
"rotation": 90
}
Then, remove the excess whitespace from the JSON object:
{"rotation":90}
Finally, append the URL-encoded version of the JSON to the end of the query string:
POST /api/v2/convert/zpl/to/zpl?params=%7B%22rotation%22%3A90%7D
Pro Tip: If you’re using Postman to construct the request, you can right-click on the parameter and perform a URL-encode or a URL-decode:
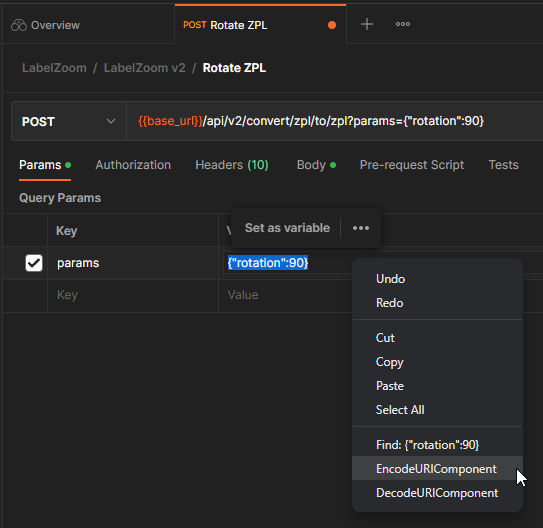
Sending the Request
Now that you have the URL finalized, the only thing left is to send a POST
to the URL where the Content-Type is text/plain
and the body is your ZPL. The response will be the rotated ZPL! How easy was that?
Code Samples
PHP with cURL
<?php
$base_url = "https://www.labelzoom.net";
$zpl = "<INSERT ZPL HERE>";
$curl = curl_init();
$params = urlencode("{\"rotation\":90}");
curl_setopt($curl, CURLOPT_RETURNTRANSFER, TRUE);
curl_setopt($curl, CURLOPT_POST, TRUE);
curl_setopt($curl, CURLOPT_HTTPHEADER, array('Content-Type: text/plain'));
curl_setopt($curl, CURLOPT_URL, "$base_url/api/v2/convert/zpl/to/zpl?params=$params");
curl_setopt($curl, CURLOPT_POSTFIELDS, $zpl);
$rotatedZPL = curl_exec($curl);
if (curl_getinfo($curl, CURLINFO_HTTP_CODE) != 200) throw new \Exception("Error converting ZPL to ZPL");
echo $rotatedZPL;